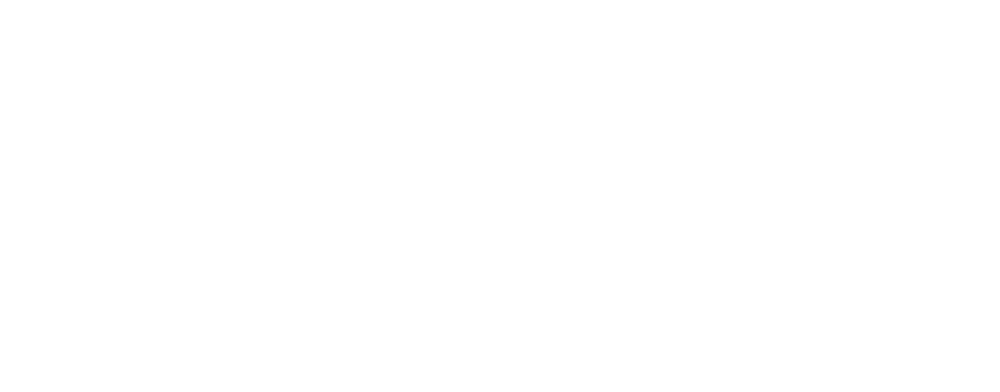
404
We can't seem to find the page you're looking for
Are you still having problems?
Please contact our support via [email protected]
We can't seem to find the page you're looking for
Are you still having problems?
Please contact our support via [email protected]